Creating Tools in edge talk
Introduction
Tools in edge talk extend your personas' capabilities by adding new functionalities. Just like personas, tools are defined in simple files that you can create and import. This guide shows you how to create your own tools using the Flight Information Tool as an example.
Tool Structure
Every edge talk tool consists of two main parts:
- The tool implementation in Shards (.shs file)
- A definition block that describes what the tool does and how to use it
Example Tool
Let's look at the Flight Information Tool, which retrieves flight details using a flight number:
; Flight Information Tool
@wire(flight-info {
; Extract flight number from input table
{Take("flight-number") | ExpectString = flight-number}
; Get API key from environment
env:aviationstack-api-key | ExpectString = api-key
; Construct API URL and make request
["https://api.aviationstack.com/v1/flights?access_key=" api-key "&flight_iata=" flight-number] | String.Join = url
none | Http.Get(url Timeout: 30) | Log("flight-info") | Await(FromJson) | ExpectTable = flight-info
flight-info:data | ExpectSeq | Limit(5) | ToString
})
; Tool definition for edge talk
{
definition: {
name: "flight_info"
description: "Get flight information for a given flight number."
parameters: {
type: "object"
properties: {
flight-number: {
type: "string"
description: "The flight number to get information for in IATA format. e.g. SQ123, no spaces."
}
}
required: ["flight-number"]
}
}
use: flight-info
}
Key Components
- Wire Definition: The main logic that processes inputs and returns results
- Tool Definition: Describes the tool's name, purpose, and required parameters
- Input Validation: Ensures inputs are the correct type and format
- Error Handling: Provides clear feedback when something goes wrong
Importing Your Tool
Once you've created your tool file, you can import it into edge talk using the same interface used for personas:
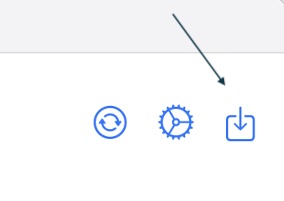
Simply click the import button and select your .shs file. edge talk will validate the tool definition and make it available to your personas.
Best Practices
- Always validate inputs using Expect* functions
- Provide clear error messages when something goes wrong
- Document your parameters thoroughly
- Keep tools focused on a single task
- Test your tool with different inputs before importing
Remember: A well-crafted tool enhances your personas' capabilities while maintaining reliability and ease of use. Take time to properly document and test your tool before importing it into edge talk.